嵌入代码
- Java
- Kotlin
- Xamarin
源代码是用 Java 编写的,用于原生 Android 开发。
第 1 步: 实施 ECC 库
在 .../android/app
的 build.gradle
中实现 ECC 库。
android {
...
defaultConfig {
...
multiDexEnabled true
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
packagingOptions {
exclude 'META-INF/DEPENDENCIES'
exclude 'META-INF/LICENSE.md'
exclude 'META-INF/NOTICE.md'
}
...
}
dependencies {
...
// Crypto
implementation 'com.nimbusds:nimbus-jose-jwt:8.9'
implementation 'org.bouncycastle:bcprov-jdk15on:1.64'
...
}
第 2 步: 私钥和公钥
第 2.1 步: 生成密钥
生成公钥和私钥。
import java.io.FileOutputStream;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import java.security.spec.ECGenParameterSpec;
import java.util.Base64;
public void generateECCKeys(String outputPath) {
try {
KeyPairGenerator kpg = KeyPairGenerator.getInstance("EC");
pg.initialize(new ECGenParameterSpec("secp256r1"), new SecureRandom());
KeyPair keyPair = kpg.generateKeyPair();
saveKeyPair(outputPath, keyPair);
} catch (Exception ex) {
ex.printStackTrace();
}
}
public void saveKeyPair(String outputPath, KeyPair keyPair) {
PrivateKey privateKey = keyPair.getPrivate();
PublicKey publicKey = keyPair.getPublic();
try {
StringBuilder content = new StringBuilder();
FileOutputStream fos = new FileOutputStream(outputPath + "./public.key");
content.append(Base64.getEncoder().encodeToString(publicKey.getEncoded()));
content.insert(0, "-----BEGIN PUBLIC KEY-----\n");
content.insert(content.length(), "\n-----END PUBLIC KEY-----");
fos.write(content.toString().getBytes());
fos.close();
content.setLength(0);
fos = new FileOutputStream(outputPath + "./private.key");
content.append(Base64.getEncoder().encodeToString(privateKey.getEncoded()));
content.insert(0, "-----BEGIN PRIVATE KEY-----\n");
content.insert(content.length(), "\n-----END PRIVATE KEY-----");
fos.write(content.toString().getBytes());
fos.close();
} catch (Exception ex) {
ex.printStackTrace();
}
}
第 2.2 步: 初始化按键
初始化将用于加密和解密过程的密钥。
TestPos(ReactApplicationContext reactContext) {
super(reactContext);
this.reactContext = reactContext;
try {
thirdAppPrivateKey = loadECPrivateKey(reactContext.getAssets().open("thirdapp_private_key.key"));
thirdAppPublicKey = loadECPublicKey(reactContext.getAssets().open("thirdapp_public_key.key"));
yippiePosPublicKey = loadECPublicKey(reactContext.getAssets().open("yippiepos_public_key.key"));
String refNum = "1.0";
secretKey = generateECDHSecret(thirdAppPrivateKey, yippiePosPublicKey, refNum);
} catch (IOException ex) {
ex.printStackTrace();
}
BA = BluetoothAdapter.getDefaultAdapter();
}
第 3 步: 加载初始化密钥
从密钥文件加载初始化的密钥。
- Private Key
- Public Key
- ECDH Secret Key
public static ECPrivateKey loadECPrivateKey(InputStream certFile) {
ECPrivateKey ecPrivateKey = null;
PrivateKey privateKey = null;
try {
byte[] encodedPrivateKey = new byte[certFile.available()];
certFile.read(encodedPrivateKey);
certFile.close();
try {
String certFileContent = new String(encodedPrivateKey)
.replace("-----BEGIN CERTIFICATE-----", "")
.replace("-----END CERTIFICATE-----","")
.replace("-----BEGIN PRIVATE KEY-----", "")
.replace("-----END PRIVATE KEY-----", "");
encodedPrivateKey = android.util.Base64.decode(certFileContent, android.util.Base64.DEFAULT);
} catch (Exception ex) {
ex.printStackTrace();
}
PKCS8EncodedKeySpec privateKeySpec = new PKCS8EncodedKeySpec(encodedPrivateKey);
KeyFactory keyFactory = KeyFactory.getInstance("EC");
privateKey = keyFactory.generatePrivate(privateKeySpec);
} catch (NoSuchAlgorithmException | InvalidKeySpecException | IOException e) {
e.printStackTrace();
}
if (privateKey instanceof ECPrivateKey){
ecPrivateKey = (ECPrivateKey) privateKey;
}
return ecPrivateKey;
}
public static ECPublicKey loadECPublicKey(InputStream certFile) {
ECPublicKey ecPublicKey = null;
PublicKey publicKey = null;
try {
byte[] publicKeyAsBytes = new byte[certFile.available()];
certFile.read(publicKeyAsBytes);
certFile.close();
try {
String certFileContent = new String(publicKeyAsBytes)
.replace("-----BEGIN CERTIFICATE-----", "")
.replace("-----END CERTIFICATE-----", "")
.replace("-----BEGIN PUBLIC KEY-----", "")
.replace("-----END PUBLIC KEY-----", "");
publicKeyAsBytes = android.util.Base64.decode(certFileContent, android.util.Base64.DEFAULT);
} catch (Exception ex) {
ex.printStackTrace();
}
X509EncodedKeySpec publicKeySpec = new X509EncodedKeySpec(publicKeyAsBytes);
KeyFactory keyFactory = KeyFactory.getInstance("EC");
publicKey = keyFactory.generatePublic(publicKeySpec);
} catch (NoSuchAlgorithmException | InvalidKeySpecException | IOException e) {
e.printStackTrace();
}
if (publicKey instanceof ECPublicKey) {
ecPublicKey = (ECPublicKey) publicKey;
}
String test = String.valueOf(publicKey);
return ecPublicKey;
}
public static SecretKey generateECDHSecret(ECPrivateKey sdkPrivateKey, ECPublicKey thirdAppPublicKey, String partyVInfo) {
SecretKey secretKey = null;
try{
secretKey = ECDH.deriveSharedSecret(thirdAppPublicKey, sdkPrivateKey, null);
if(partyVInfo != null){
ConcatKDF kdf = new ConcatKDF("SHA-256");
int length = ECDH.sharedKeyLength(JWEAlgorithm.ECDH_ES,
EncryptionMethod.A128CBC_HS256);
if(partyVInfo.isEmpty()){
secretKey = kdf.deriveKey(secretKey, length, null, null, null, null, null);
} else {
secretKey = kdf.deriveKey(
secretKey,
length,
ConcatKDF.encodeDataWithLength(new byte[0]),
ConcatKDF.encodeDataWithLength(new byte[0]),
ConcatKDF.encodeDataWithLength(partyVInfo.getBytes()),
ConcatKDF.encodeIntData(length),
ConcatKDF.encodeNoData());
}
}
} catch (Exception ex) {
ex.printStackTrace();
}
return secretKey;
}
第 4 步: 声明输入
声明请求中的所有输入参数并创建 JSON 字符串。
- 无效适用于结算前或同一天内的交易。
- 退款适用于结算后或超过一天的交易
- DuitNow QR 付款仅适用于退款。
- 无效
- 退款
public void receiveMessage(ReadableMap data) {
String msgVer = data.getString("msgVer");
String callerDevice = data.getString("callerDevice");
String callerDeviceVer = data.getString("callerDeviceVer");
String txnID = UUID.randomUUID().toString().replaceAll("-", "").toUpperCase();
String txnDateTime = data.getString("txnDateTime");
String email = data.getString("email");
String password = data.getString("password");
String crcyCde = data.getString("crcyCde");
String amt = data.getString("amt");
String stan = data.getString("stan");
String authIdResp = data.getString("authIdResp");
String rrn = data.getString("rrn");
String mrn = data.getString("mrn");
String jsonMessage = "{'TxnTyp' : '" + TestPos.TransactionType.VOD +
"', 'MsgVer' : '" + msgVer +
"', 'CallerDeviceType' : '" + callerDevice +
"', 'CallerDeviceVer' : '" + callerDeviceVer +
"', 'TxnID' : '" + txnID +
"', 'LocalTxnDTTime' : '" + txnDateTime +
"', 'Email' : '" + email +
"', 'Pwd' : '" + password +
"', 'AmtTxn' : '" + amt +
"', 'CrcyTxn' : '" + crcyCde +
"', 'STAN' : '" + stan +
"', 'AuthIdResp' : '" + authIdResp +
"', 'RRN' : '" + rrn +
"', 'MRN' : '" + mrn + "'}";
ECKey publicKeyASJWK = new ECKey.Builder(Curve.P_256, thirdAppPublicKey)
.keyID(UUID.randomUUID().toString())
.build();
String jsonMessageEncrypted = encryptWithFormatJWE(txnID,
publicKeyASJWK.toJSONString(), secretKey, JWEAlgorithm.DIR, jsonMessage);
startActivity(REQ_SALE, jsonMessageEncrypted);
}
public void receiveMessage(ReadableMap data) {
String msgVer = data.getString("msgVer");
String callerDevice = data.getString("callerDevice");
String callerDeviceVer = data.getString("callerDeviceVer");
String refundTxnID = UUID.randomUUID().toString().replaceAll("-", "").toUpperCase();
String oriTxnID = data.getString("oriTxnID");
String txnDateTime = data.getString("txnDateTime");
String email = data.getString("email");
String password = data.getString("password");
String crcyCde = data.getString("crcyCde");
String amt = data.getString("amt");
String jsonMessage = "{'MsgVer' : '" + msgVer +
"', 'CallerDeviceType' : '" + callerDevice +
"', 'CallerDeviceVer' : '" + callerDeviceVer +
"', 'RefundTxnID' : '" + refundTxnID +
"', 'OriTxnID' : '" + oriTxnID +
"', 'LocalTxnDTTime' : '" + txnDateTime +
"', 'Email' : '" + email +
"', 'Pwd' : '" + password +
"', 'RefundAmt' : '" + amt +
"', 'CrcyTxn' : '" + crcyCde + "'}";
ECKey publicKeyASJWK = new ECKey.Builder(Curve.P_256, thirdAppPublicKey)
.keyID(UUID.randomUUID().toString())
.build();
String jsonMessageEncrypted = encryptWithFormatJWE(txnID,
publicKeyASJWK.toJSONString(), secretKey, JWEAlgorithm.DIR, jsonMessage);
startActivity(REQ_SALE, jsonMessageEncrypted);
}
第 5 步: 加密请求消息
在将请求消息发送到 Kayaaku POS 应用程序之前对其进行加密。
public static String encryptWithFormatJWE(String transactionID, String sdkPublicKeyAsJWK, SecretKey secretKey, JWEAlgorithm jweAlgorithm, String message) {
String encryptedMessage = "";
try {
//header
JWEHeader.Builder builder = new JWEHeader.Builder(jweAlgorithm, EncryptionMethod.A128CBC_HS256);
if (!TextUtils.isEmpty(transactionID)) {
builder.keyID(transactionID);
}
if (!TextUtils.isEmpty(sdkPublicKeyAsJWK)) {
builder.ephemeralPublicKey(ECKey.parse(sdkPublicKeyAsJWK));
}
JWEHeader header = builder.build();
//encrypt
if(jweAlgorithm == JWEAlgorithm.DIR) {
Provider bouncyCastleProvider = BouncyCastleProviderSingleton.getInstance();
Security.addProvider((bouncyCastleProvider));
JWEObject jweObject = new JWEObject(header, new Payload(message));
jweObject.encrypt(new DirectEncrypter(secretKey));
encryptedMessage = jweObject.serialize();
} else if (jweAlgorithm == JWEAlgorithm.ECDH_ES) {
// build JWE structure
JWECryptoParts jweParts = ContentCryptoProvider.encrypt(header, message.getBytes(), secretKey, null, new JWEJCAContext());
// JWE Protected Header
StringBuilder stringBuilder = new StringBuilder(header.toBase64URL().toString());
stringBuilder.append('.');
// Encrypted Key
if (jweParts.getEncryptedKey() != null) {
stringBuilder.append(jweParts.getEncryptedKey().toString());
}
stringBuilder.append('.');
// Initialization Vector
if (jweParts.getInitializationVector() != null) {
stringBuilder.append(jweParts.getInitializationVector().toString());
}
stringBuilder.append('.');
// Ciphertext
stringBuilder.append(jweParts.getCipherText().toString());
stringBuilder.append('.');
// Authentication Tag
if (jweParts.getAuthenticationTag() != null) {
stringBuilder.append(jweParts.getAuthenticationTag().toString());
}
encryptedMessage = stringBuilder.toString();
} else {
Log.d("Tag", "Unsupported other algorithms");
}
} catch (Exception ex) {
ex.printStackTrace();
}
return encryptedMessage;
}
第 6 步: 执行应用程序到应用程序的调用
商家可以选择使用INTENT或蓝牙来执行应用程序到应用程序的通信。
第 6.1 步: 使用 INTENT 的应用到应用
要使用 INTENT,Kayaaku POS 应用程序和商家的 POS 应用程序必须安装在同一设备上。
按照下面的示例代码使用startActivityForResult()
。
// start intent activity
private void startActivity (int messageType, String encryptedMessage) {
if (messageType == REQ_SALE) {
Intent intent = new Intent(ACTION_SALE); // ACTION_SALE = "com.finexuscards.yippiepos.xxx.SALES"
intent.putExtra("Data", encryptedMessage);
startActivityForResult(intent, REQ_SALE); // REQ_SALE = 0
} else if (messageType == REQ_VOID) {
Intent intent = new Intent(ACTION_VOID); // ACTION_VOID = "com.finexuscards.yippiepos.xxx.VOID"
intent.putExtra("Data", encryptedMessage);
startActivityForResult(intent, REQ_VOID); // REQ_VOID = 1
} else if (messageType == REQ_REFUND) {
Intent intent = new Intent(ACTION_REFUND); // ACTION_VOID = "com.finexuscards.yippiepos.xxx.REFUND"
intent.putExtra("Data", encryptedMessage);
startActivityForResult(intent, REQ_REFUND); // REQ_REFUND = 2
}
}
// get response from onActivityResult()
protected void onActivityResult(int requestCode,int resultCode,Intent data){
super.onActivityResult(requestCode,resultCode,data);
String encryptedResponse = data.getStringExtra("Data");
}
第 6.2 步: 使用蓝牙的应用程序到应用程序
按照下面的示例代码使用startActivityForResult()
。
Import android.bluetooth.BluetoothSocket;
private BluetoothSocket mBluetoothSocket;
// send request via bluetooth
public void sendMessage(String message) {
OutputStream outputStream = mBluetoothSocket.getOutputStream();
outputStream.write((encryptedMessage + “\n”).getBytes());
}
// receive response via Bluetooth
private void startListener() {
InputStream inputStream = mBluetoothSocket.getInputStream();
bytes[] buffer = new byte[1024];
int bytes;
bytes = inputStream.read(buffer);
String encryptedResponse = new String(buffer, 0, bytes);
}
第 7 步: 解密响应消息
使用decryptWithFormatJWE
函数解密从 Kayaaku POS 应用程序检索到的响应消息。
public static String decryptWithFormatJWE(SecretKey secretKey, String message) {
String decryptedMessage = "";
try {
// parse message to Object
JWEObject jweObject = JWEObject.parse(message);
// get header
JWEAlgorithm algorithm = jweObject.getHeader().getAlgorithm();
EncryptionMethod encryptionMethod = jweObject.getHeader().getEncryptionMethod();
// decrypt
if (algorithm.equals(JWEAlgorithm.DIR)) {
if (encryptionMethod == EncryptionMethod.A128CBC_HS256 || encryptionMethod == EncryptionMethod.A128GCM) {
Provider bouncyCastleProvider = BouncyCastleProviderSingleton.getInstance();
Security.addProvider(bouncyCastleProvider);
// start decrypting
jweObject.decrypt(new DirectDecrypter(secretKey));
decryptedMessage = jweObject.getPayload().toString();
} else {
Log.d("Tag", "Unsupported other encryption method:" + encryptionMethod);
}
} else {
Log.d("Tag", "Unsupported other algorithms");
}
} catch (Exception ex) {
Log.e("Tag", "decryptedMessage Failed:" + ex.getMessage());
ex.printStackTrace();
}
return decryptedMessage;
}
第 1 步: 实施 ECC 库
implementation("com.nimbusds:nimbus-jose-jwt:8.9")
implementation("org.bouncycastle:bcprov-jdk15on:1.64")
第 2 步: 私钥和公钥
第 2.1 步: 生成密钥
生成用于安全通信的公钥和私钥。
import java.io.FileOutputStream;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import java.security.spec.ECGenParameterSpec;
import java.util.Base64;
public void generateECCKeys(String outputPath) {
try {
KeyPairGenerator kpg = KeyPairGenerator.getInstance("EC");
pg.initialize(new ECGenParameterSpec("secp256r1"), new SecureRandom());
KeyPair keyPair = kpg.generateKeyPair();
saveKeyPair(outputPath, keyPair);
} catch (Exception ex) {
ex.printStackTrace();
}
}
public void saveKeyPair(String outputPath, KeyPair keyPair) {
PrivateKey privateKey = keyPair.getPrivate();
PublicKey publicKey = keyPair.getPublic();
try {
StringBuilder content = new StringBuilder();
FileOutputStream fos = new FileOutputStream(outputPath + "./public.key");
content.append(Base64.getEncoder().encodeToString(publicKey.getEncoded()));
content.insert(0, "-----BEGIN PUBLIC KEY-----\n");
content.insert(content.length(), "\n-----END PUBLIC KEY-----");
fos.write(content.toString().getBytes());
fos.close();
content.setLength(0);
fos = new FileOutputStream(outputPath + "./private.key");
content.append(Base64.getEncoder().encodeToString(privateKey.getEncoded()));
content.insert(0, "-----BEGIN PRIVATE KEY-----\n");
content.insert(content.length(), "\n-----END PRIVATE KEY-----");
fos.write(content.toString().getBytes());
fos.close();
} catch (Exception ex) {
ex.printStackTrace();
}
}
第 2.2 步: 初始化按键
初始化将用于加密和解密过程的密钥。
constructor(reactContext: ReactApplicationContext) : super(reactContext) {
this.reactContext = reactContext
try {
thirdAppPrivateKey = loadECPrivateKey(reactContext.getAssets().open("thirdapp_private_key.key"))
thirdAppPublicKey = loadECPublicKey(reactContext.getAssets().open("thirdapp_public_key.key"))
yippiePosPublicKey = loadECPublicKey(reactContext.getAssets().open("yippiepos_public_key.key"))
val refNum = "1.0"
secretKey = generateECDHSecret(thirdAppPrivateKey, yippiePosPublicKey, refNum)
} catch (ex: IOException) {
ex.printStackTrace()
}
BA = BluetoothAdapter.getDefaultAdapter()
}
第 3 步: 加载初始化密钥
从密钥文件加载初始化的密钥。
- Private Key
- Public Key
- ECDH Secret Key
fun loadECPrivateKey(certFile: InputStream): ECPrivateKey {
var ecPrivateKey: ECPrivateKey? = null
var privateKey: PrivateKey? = null
try {
val encodedPrivateKey = ByteArray(certFile.available())
certFile.read(encodedPrivateKey)
certFile.close()
try {
val certFileContent = String(encodedPrivateKey)
.replace("-----BEGIN CERTIFICATE-----", "")
.replace("-----END CERTIFICATE-----", "")
.replace("-----BEGIN PRIVATE KEY-----", "")
.replace("-----END PRIVATE KEY-----", "")
encodedPrivateKey = android.util.Base64.decode(certFileContent, android.util.Base64.DEFAULT)
} catch (ex: Exception) {
ex.printStackTrace()
}
val privateKeySpec = PKCS8EncodedKeySpec(encodedPrivateKey)
val keyFactory = KeyFactory.getInstance("EC")
privateKey = keyFactory.generatePrivate(privateKeySpec)
} catch (NoSuchAlgorithmException | InvalidKeySpecException | IOException e) {
e.printStackTrace()
}
if (privateKey is ECPrivateKey) {
ecPrivateKey = privateKey
}
return ecPrivateKey
}
fun loadECPublicKey(certFile: InputStream): ECPublicKey {
var ecPublicKey: ECPublicKey? = null
var publicKey: PublicKey? = null
try {
val encodedPublicKey = ByteArray(certFile.available())
certFile.read(encodedPublicKey)
certFile.close()
try {
val certFileContent = String(encodedPublicKey)
.replace("-----BEGIN CERTIFICATE-----", "")
.replace("-----END CERTIFICATE-----", "")
.replace("-----BEGIN PUBLIC KEY-----", "")
.replace("-----END PUBLIC KEY-----", "")
encodedPublicKey = android.util.Base64.decode(certFileContent, android.util.Base64.DEFAULT)
} catch (ex: Exception) {
ex.printStackTrace()
}
val publicKeySpec = X509EncodedKeySpec(encodedPublicKey)
val keyFactory = KeyFactory.getInstance("EC")
publicKey = keyFactory.generatePublic(publicKeySpec)
} catch (NoSuchAlgorithmException | InvalidKeySpecException | IOException e) {
e.printStackTrace()
}
if (publicKey is ECPublicKey) {
ecPublicKey = publicKey
}
return ecPublicKey
}
fun generateECDHSecret(sdkPrivateKey: ECPrivateKey,
thirdAppPublicKey: ECPublicKey,
partyVInfo: String): SecretKey {
var secretKey: SecretKey? = null
try {
secretKey = ECDH.deriveSharedSecret(thirdAppPublicKey, sdkPrivateKey, null)
if (partyVInfo != null) {
val kdf = ConcatKDF("SHA-256")
val length = ECDH.sharedKeyLength(JWEAlgorithm.ECDH_ES,
EncryptionMethod.A128CBC_HS256)
if (partyVInfo.isEmpty()) {
secretKey = kdf.deriveKey(
secretKey,
length,
null,
null,
null,
null,
null)
} else {
secretKey = kdf.deriveKey(
secretKey,
length,
ConcatKDF.encodeDataWithLength(new byte[0]),
ConcatKDF.encodeDataWithLength(new byte[0]),
ConcatKDF.encodeDataWithLength(partyVInfo.toByteArray()),
ConcatKDF.encodeIntData(length),
ConcatKDF.encodeNoData())
}
}
} catch (ex: Exception) {
ex.printStackTrace()
}
return secretKey
}
第 4 步: 声明输入
声明请求中的所有输入参数并创建 JSON 字符串。
- 无效适用于结算前或同一天内的交易。
- 退款适用于结算后或超过一天的交易
- DuitNow QR 付款仅适用于退款。
- 无效
- 退款
fun receiveMessage(data: ReadableMap) {
val msgVer = data.getString("msgVer")
val callerDevice = data.getString("callerDevice")
val callerDeviceVer = data.getString("callerDeviceVer")
val txnID = UUID.randomUUID().toString().replaceAll("-", "").toUpperCase()
val txnDateTime = data.getString("txnDateTime")
val email = data.getString("email")
val password = data.getString("password")
val crcyCde = data.getString("crcyCde")
val amt = data.getString("amt")
val stan = data.getString("stan")
val authIdResp = data.getString("authIdResp")
val rrn = data.getString("rrn")
val mrn = data.getString("mrn")
val jsonMessage = """
{
"TxnTyp": "$TestPos.TransactionType.VOD",
"MsgVer": "$msgVer",
"CallerDeviceType": "$callerDevice",
"CallerDeviceVer": "$callerDeviceVer",
"TxnID": "$txnID",
"LocalTxnDTTime": "$txnDateTime",
"Email": "$email",
"Pwd": "$password",
"AmtTxn": "$amt",
"CrcyTxn": "$crcyCde",
"STAN": "$stan",
"AuthIdResp": "$authIdResp",
"RRN": "$rrn",
"MRN": "$mrn"
}
""".trimIndent()
fun receiveMessage(data: ReadableMap) {
val msgVer = data.getString("msgVer")
val callerDevice = data.getString("callerDevice")
val callerDeviceVer = data.getString("callerDeviceVer")
val refundTxnID = UUID.randomUUID().toString().replaceAll("-", "").toUpperCase()
val oriTxnID = data.getString("oriTxnID")
val txnDateTime = data.getString("txnDateTime")
val email = data.getString("email")
val password = data.getString("password")
val crcyCde = data.getString("crcyCde")
val amt = data.getString("amt")
val jsonMessage = """
{
"MsgVer": "$msgVer",
"CallerDeviceType": "$callerDevice",
"CallerDeviceVer": "$callerDeviceVer",
"RefundTxnID": "$refundTxnID",
"OriTxnID": "$oriTxnID",
"LocalTxnDTTime": "$txnDateTime",
"Email": "$email",
"Pwd": "$password",
"RefundAmt": "$amt",
"CrcyTxn": "$crcyCde"
}
""".trimIndent()
第 5 步: 加密字符串
获取字符串形式的jsonMessage
后,使用encryptWithFormatJWE
函数对其进行加密。
val publicKeyASJWK = ECKey.newBuilder(Curve.P_256, thirdAppPublicKey)
.keyID(UUID.randomUUID().toString())
.build()
val jsonMessageEncrypted = encryptWithFormatJWE(txnID,
publicKeyASJWK.toJSONString(), secretKey, JWEAlgorithm.DIR, jsonMessage)
}
第 6 步: 执行应用程序到应用程序的调 用
商家可以选择使用INTENT或蓝牙来执行应用程序到应用程序的通信。
第 6.1 步: 使用 INTENT 的应用到应用
要使用 INTENT,Kayaaku POS 应用程序和商家的 POS 应用程序必须安装在同一设备上。
按照下面的示例代码使用startActivityForResult()
。
if (mMessageType == REQ_SALE) {
val intent = Intent(ACTION_SALE)
intent.putExtra("Data", encryptedMessage)
startActivityForResult(intent, REQ_SALE)
} else if (mMessageType == REQ_VOID) {
val intent = Intent(ACTION_VOID)
intent.putExtra("Data", encryptedMessage)
startActivityForResult(intent, REQ_VOID)
} else if (mMessageType == REQ_REFUND) {
val intent = Intent(ACTION_REFUND)
intent.putExtra("Data", encryptedMessage)
startActivityForResult(intent, REQ_REFUND)
}
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
val encryptedResponse = data?.getStringExtra("Data")
}
第 6.2 步: 使用蓝牙的应用程序到应用程序
val outputStream = bluetoothSocket.getOutputStream()
outputStream.write((encryptedMessage + "\n").toByteArray())
val inputStream = bluetoothSocket.getInputStream()
val bytes = inputStream.read()
val response = String(bytes)
第 7 步: 加密请求消息
使用encryptWithFormatJWE
函数对要发送到 Kayaaku POS 应用程序的请求消息进行加密。
fun encryptWithFormatJWE(transactionID: String, sdkPublicKeyAsJWK: String, secretKey: SecretKey, jweAlgorithm: JWEAlgorithm, message: String): String {
val encryptedMessage = ""
try {
val builder = JWEHeader.Builder(jweAlgorithm, EncryptionMethod.A128CBC_HS256)
if (!TextUtils.isEmpty(transactionID)) {
builder.keyID(transactionID)
}
if (!TextUtils.isEmpty(sdkPublicKeyAsJWK)) {
builder.ephemeralPublicKey(ECKey.parse(sdkPublicKeyAsJWK))
}
val header = builder.build()
if (jweAlgorithm == JWEAlgorithm.DIR) {
val bouncyCastleProvider = BouncyCastleProviderSingleton.getInstance()
Security.addProvider(bouncyCastleProvider)
val jweObject = JWEObject(header, Payload(message))
jweObject.encrypt(DirectEncrypter(secretKey))
encryptedMessage = jweObject.serialize()
} else if (jweAlgorithm == JWEAlgorithm.ECDH_ES) {
val jweParts = ContentCryptoProvider.encrypt(header, message.toByteArray(),
secretKey, null, JWEJCAContext())
val stringBuilder = StringBuilder(header.toBase64URL().toString())
stringBuilder.append('.')
if (jweParts.getEncryptedKey() != null) {
stringBuilder.append(jweParts.getEncryptedKey().toString())
}
stringBuilder.append('.')
if (jweParts.getInitializationVector() != null) {
stringBuilder.append(jweParts.getInitializationVector().toString())
}
stringBuilder.append('.')
stringBuilder.append(jweParts.getCipherText().toString())
stringBuilder.append('.')
if (jweParts.getAuthenticationTag() != null) {
stringBuilder.append(jweParts.getAuthenticationTag().toString())
}
encryptedMessage = stringBuilder.toString()
} else {
Log.d("Tag", "Unsupported other algorithms")
}
}
第 8 步: 解密响应消息
使用decryptWithFormatJWE
函数解密从 Kayaaku POS 应用程序检索到的响应消息。
fun decryptWithFormatJWE(secretKey: SecretKey, message: String): String {
val decryptedMessage = ""
try {
// parse message to Object
val jweObject = JWEObject.parse(message)
// get header
val algorithm = jweObject.getHeader().getAlgorithm()
val encryptionMethod = jweObject.getHeader().getEncryptionMethod()
// decrypt
if (algorithm.equals(JWEAlgorithm.DIR)) {
if (encryptionMethod == EncryptionMethod.A128CBC_HS256
|| encryptionMethod == EncryptionMethod.A128GCM) {
val bouncyCastleProvider = BouncyCastleProviderSingleton.getInstance()
Security.addProvider(bouncyCastleProvider)
//// start decrypting
jweObject.decrypt(new DirectDecrypter(secretKey))
decryptedMessage = jweObject.getPayload().toString()
} else {
Log.d("Tag", "Unsupported other encryption method:" + encryptionMethod)
}
} else {
Log.d("Tag", "Unsupported other algorithms")
}
} catch (Exception ex) {
Log.e("Tag", "decryptedMessage Failed:" + ex.getMessage())
ex.printStackTrace()
}
return decryptedMessage
}
第 1 步: 下载库
- System.IdentityModel.Tokens.Jwt 由 Microsoft

- BouncyCastle.Cryptography 由 Legion of the Bouncy Castle Inc. 提供

- 何塞-twt 作者:Dmitry Vsekhvalnov
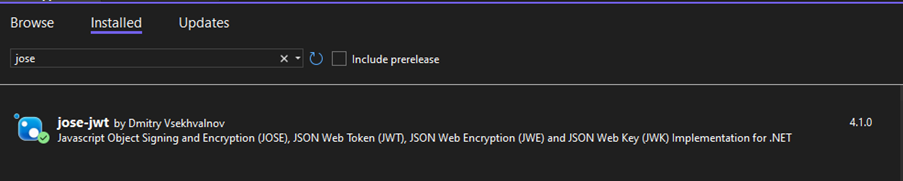
第 2 步: 私钥和公钥
生成公钥和私钥。
第 2.1 步: 生成密钥 (Java)
import java.io.FileOutputStream;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import java.security.spec.ECGenParameterSpec;
import java.util.Base64;
public void generateECCKeys(String outputPath) {
try {
KeyPairGenerator kpg = KeyPairGenerator.getInstance("EC");
pg.initialize(new ECGenParameterSpec("secp256r1"), new SecureRandom());
KeyPair keyPair = kpg.generateKeyPair();
saveKeyPair(outputPath, keyPair);
} catch (Exception ex) {
ex.printStackTrace();
}
}
public void saveKeyPair(String outputPath, KeyPair keyPair) {
PrivateKey privateKey = keyPair.getPrivate();
PublicKey publicKey = keyPair.getPublic();
try {
StringBuilder content = new StringBuilder();
FileOutputStream fos = new FileOutputStream(outputPath + "./public.key");
content.append(Base64.getEncoder().encodeToString(publicKey.getEncoded()));
content.insert(0, "-----BEGIN PUBLIC KEY-----\n");
content.insert(content.length(), "\n-----END PUBLIC KEY-----");
fos.write(content.toString().getBytes());
fos.close();
content.setLength(0);
fos = new FileOutputStream(outputPath + "./private.key");
content.append(Base64.getEncoder().encodeToString(privateKey.getEncoded()));
content.insert(0, "-----BEGIN PRIVATE KEY-----\n");
content.insert(content.length(), "\n-----END PRIVATE KEY-----");
fos.write(content.toString().getBytes());
fos.close();
} catch (Exception ex) {
ex.printStackTrace();
}
}
第 2.2 步: 初始化按键
初始化将用于加密和解密过程的密钥。
ECPrivateKey thirdAppPrivateKey = loadECPrivateKey(assets.Open("thirdapp_private_key.key"));
ECPublicKey thirdAppPublicKey = loadECPublicKey(assets.Open("thirdapp_public_key.key"));
ECPublicKey yippiePosPublicKey = loadECPublicKey(assets.Open("yippiepos_public_key.key"));
第 3 步: 加载初始化密钥
从密钥文件加载初始化的密钥。
- Private Key
- Public Key
- ECDH Secret Key
public static ECPrivateKey loadECPrivateKey(System.IO.Stream certFile)
{
ECPrivateKey ecPrivateKey = null;
try {
MemoryStream memoryStream = new MemoryStream();
certFile.CopyTo(memoryStream);
byte[] encodedPrivateKey = memoryStream.ToArray();
try {
string certFileContent = System.Text.Encoding.UTF8.GetString(encodedPrivateKey)
.Replace("-----BEGIN CERTIFICATE-----", "")
.Replace("-----END CERTIFICATE-----", "")
.Replace("-----BEGIN PRIVATE KEY-----", "")
.Replace("-----END PRIVATE KEY-----", "");
encodedPrivateKey = Convert.FromBase64String(certFileContent);
}
catch (Exception ex) {
//to do …
}
PKCS8EncodedKeySpec privateKeySpec = new PKCS8EncodedKeySpec(encodedPrivateKey);
KeyFactory keyFactory = KeyFactory.GetInstance("EC");
ecPrivateKey = (ECPrivateKey) keyFactory.GeneratePrivate(privateKeySpec);
}
catch (Exception e) {
//to do …
}
return ecPrivateKey;
}
public static ECPublicKey loadECPublicKey(System.IO.Stream certFile)
{
ECPublicKey ecPublicKey = null;
try
{
MemoryStream memoryStream = new MemoryStream();
certFile.CopyTo(memoryStream);
byte[] publicKeyAsBytes = memoryStream.ToArray();
try
{
string certFileContent = System.Text.Encoding.UTF8.GetString(publicKeyAsBytes)
.Replace("-----BEGIN CERTIFICATE-----", "")
.Replace("-----END CERTIFICATE-----", "")
.Replace("-----BEGIN PUBLIC KEY-----", "")
.Replace("-----END PUBLIC KEY-----", "");
publicKeyAsBytes = Convert.FromBase64String(certFileContent);
}
catch (Exception ex)
{
//to do …
}
X509EncodedKeySpec publicKeySpec = new X509EncodedKeySpec(publicKeyAsBytes);
KeyFactory keyFactory = KeyFactory.GetInstance("EC");
ecPublicKey = (ECPublicKey) keyFactory.GeneratePublic(publicKeySpec);
}
catch (Exception e) {
//to do …
}
return ecPublicKey;
}
密钥仅与 Finexus 公钥和商家私钥一起生成一次。
byte[] encoded = Base64.getDecoder().decode("MEECAQ…"); // thirdapp_private_key
KeyFactory kf = KeyFactory.getInstance("EC");
EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(encoded);
ECPrivateKey privateKey = (ECPrivateKey) kf.generatePrivate(keySpec);
byte[] encodedFNX = Base64.getDecoder().decode("MFkwEwYHDAQc…"); //yippiepos_public_key
KeyFactory kfFNX = KeyFactory.getInstance("EC");
EncodedKeySpec keySpecFNX = new X509EncodedKeySpec(encodedFNX);
ECPublicKey publicKey = (ECPublicKey) kfFNX.generatePublic(keySpecFNX);
SecretKey secretKey = generateECDHSecret(privateKey,publicKey,"1.0");
StringBuilder contentSecret = new StringBuilder();
contentSecret.append(Base64.getEncoder().encodeToString(secretKey.getEncoded()));
FileOutputStream fos = new FileOutputStream(outputPath + "./secret.key");
fos.write(contentSecret.toString().getBytes());
fos.close();